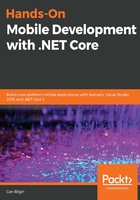
Master/detail view
On Android and UWP, the prominent navigation pattern and the associated page type is master/detail, using a so-called navigation drawer. In this pattern, jumping (across the different tiers of the hierarchy) or cross-navigating (within the same tier) across the navigation structure is maintained with ContentPage, which is known as the master page. User interaction with the master page, which is displayed in the navigation drawer, is propagated to the Detail view. In this setup, the navigation stack exists for the detail view, whilst the master view is static.
In order to replicate the tab structure in the previous example, we can create MasterDetailPage, which will host the list of menu items. MasterDetailPage will consist of the Master content page and the Detail page, which will host NavigationPage to create the navigation stack.
The Master page could look as follows:
<MasterDetailPage.Master>
<ContentPage Title="Main" Padding="0,60,0,0" Icon="slideout.png">
<StackLayout>
<ListView
x:Name="listView"
ItemsSource="{Binding .}"
SeparatorVisibility="None">
<ListView.ItemTemplate>
<DataTemplate>
<ViewCell>
<Grid Padding="5,10">
<Grid.ColumnDefinitions>
<ColumnDefinition Width="30"/>
<ColumnDefinition Width="*" />
</Grid.ColumnDefinitions>
<Image Source="{Binding Icon}" />
<Label Grid.Column="1" Text="{Binding
Title}" />
</Grid>
</ViewCell>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</StackLayout>
</ContentPage>
</MasterDetailPage.Master>
Notice that the Master page simply creates a ListView with the menu item entries. It is also important to note that the so-called hamburger menu icon needs to be added as the Icon property for the Master page (see slideout.png), otherwise the title of the master page is used instead of the menu icon.
The details page assignment would then look as follows:
<MasterDetailPage.Detail>
<NavigationPage Title="List">
<x:Arguments>
<local:ListItemView />
</x:Arguments>
</NavigationPage>
</MasterDetailPage.Detail>
Now, running the application would create the navigation drawer and the contained Master page:

To complete the implementation, we also need to handle the ItemTapped event from the master list:
private void Handle_ItemTapped(object sender, Xamarin.Forms.ItemTappedEventArgs e)
{
if(e.Item is NavigationItem item)
{
Page detailPage = null;
// Removed for brevity - Initialization of detailPage
this.Detail = new NavigationPage(detailPage);
this.IsPresented = false;
}
}
Now, the implementation is complete. Every time a menu item is used, the navigation category is changed and a new navigation stack is created; however, within a navigation category, the navigation stack is intact. Also, note that the IsPresented property of the MasterDetailPage is set to false to dismiss the master page immediately after a new detail view is created.