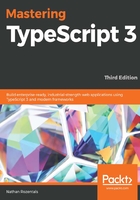
The let keyword
Variables in JavaScript are defined by using the var keyword. Unfortunately, the JavaScript runtime is very lenient on where these definitions occur, and will allow a variable to be used before it has been defined. If the JavaScript runtime comes across a variable that has not been previously defined, or given a value, then the value for this variable will be undefined. Consider the following code:
console.log(`anyValue = ${anyValue}`); var anyValue = 2; console.log(`anyValue = ${anyValue}`);
Here, we start by logging the value of a variable named anyValue to the console. Note, however, that the anyValue variable is only defined on the second line of this code snippet. In other words, we can use a variable in JavaScript before it is defined. The output of this code is as follows:
anyValue = undefined anyValue = 2
The semantics of using the var keyword presents us with a small problem. Using the var keyword does not check to see whether the variable itself has been defined before we actually use it. This could obviously lead to unwanted behavior, since the value of an undefined or unallocated variable is always undefined.
TypeScript introduces the let keyword that can be used in the place of the var keyword when defining variables. One of the advantages of using the let keyword is that we cannot use a variable name before it has been defined. Consider the following code:
console.log(`letValue = ${lValue}`); let lValue = 2;
Here, we are attempting to log the value of the variable lValue to the console, even before it has been defined, similar to how we were using the anyValue variable earlier. However, when using the let keyword instead of the var keyword, this code will generate an error, as follows:
error TS2448: Block-scoped variable 'lValue'' used before its declaration.
To fix this code, then, we need to define our lValue variable before it is first used, as follows:
let lValue = 2; console.log(`lValue = ${lValue}`);
This code will compile correctly, and output the following to the console:
lValue = 2
Another side effect of using the let keyword is that variables defined with let are block-scoped. This means that their value and definition are limited to the block of code that they reside in. As an example of this, consider the following code:
let lValue = 2; console.log(`lValue = ${lValue}`); if (lValue == 2) { let lValue = 2001; console.log(`block scoped lValue : ${lValue} `); } console.log(`lValue = ${lValue}`);
Here, we define the lValue variable on the first line using the let keyword, and assign a value of 2 to it. We then log the value of lValue to the console. On the first line within the if statement, note how we are redefining a variable named lValue to hold the value 2001. We are then logging the value of lValue to the console (within the if statement block). The last line of this code snippet again logs the value of the lValue variable to the console, but this time, lValue is outside of the if statement block-scope. The output of this code is as follows:
lValue = 2 block scoped lValue : 2001 lValue = 2
What these results demonstrate is that let variables are confined to the scope in which they are defined. In other words, the let lValue = 2001; statement defines a new variable that will only be visible inside the if statement block of code. As it is a new variable, it will also not influence the value of the lValue variable that is outside of its scope. This is why the value of lValue is 2 both before and after the if statement block, and 2001 within it.
The let statement, therefore, provides us with a safer way of declaring variables and limiting their validity to the current scope.