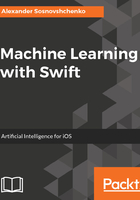
上QQ阅读APP看书,第一时间看更新
Evaluating performance of the model on iOS
I'm not describing here a .csv parsing in Swift; if you are interested in the details, please see the supplementary materials. Assuming that you've successfully loaded the test data in the form of two arrays, [Double] for features and [String] for labels, have a go at the following code:
let (xMat, yVec) = loadCSVData()
To create a decision tree and evaluate it, try this:
let sklDecisionTree = DecisionTree() let xSKLDecisionTree = xMat.map { (x: [Double]) -> DecisionTreeInput in return DecisionTreeInput(length: x[0], fluffy: x[1], color_light_black: x[2], color_pink_gold: x[3], color_purple_polka_dot: x[4], color_space_gray: x[5]) } let predictionsSKLTree = try! xSKLDecisionTree .map(sklDecisionTree.prediction) .map{ prediction in return prediction.label == "rabbosaurus" ? 0 : 1 } let groundTruth = yVec.map{ $0 == "rabbosaurus" ? 0 : 1 } let metricsSKLDecisionTree = evaluateAccuracy(yVecTest: groundTruth, predictions: predictionsSKLTree) print(metricsSKLDecisionTree)
To create a random forest and evaluate it, trying using the following code:
let sklRandomForest = RandomForest() let xSKLRandomForest = xMat.map { (x: [Double]) -> RandomForestInput in return RandomForestInput(length: x[0], fluffy: x[1], color_light_black: x[2], color_pink_gold: x[3], color_purple_polka_dot: x[4], color_space_gray: x[5]) } let predictionsSKLRandomForest = try! xSKLRandomForest.map(sklRandomForest.prediction).map{$0.label == "rabbosaurus" ? 0 : 1} let metricsSKLRandomForest = evaluateAccuracy(yVecTest: groundTruth, predictions: predictionsSKLRandomForest) print(metricsSKLRandomForest)
This is an example of how you can evaluate your model's prediction quality in the Swift application. The structure, that contains the results of evaluation is as follows:
struct Metrics: CustomStringConvertible { let confusionMatrix: [[Int]] let normalizedConfusionMatrix: [[Double]] let accuracy: Double let precision: Double let recall: Double let f1Score: Double var description: String { return """ Confusion Matrix: (confusionMatrix) Normalized Confusion Matrix: (normalizedConfusionMatrix) Accuracy: (accuracy) Precision: (precision) Recall: (recall) F1-score: (f1Score) """ } }
For the function for quality assessment, here's the code:
func evaluateAccuracy(yVecTest: [Int], predictions: [Int]) -> Metrics {