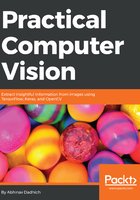
Introduction to filters
Filters are operations on an image to modify them so that they are usable for other computer vision tasks or to give us required information. These perform various functions such as removing noise from an image, extracting edges in an image, blurring an image, removing unwanted objects etc. We will see their implementations and understand the results.
Filtering techniques are necessary because there are several factors that may lead to noise in an image or undesired information in an image. Taking a picture in sunlight, induces lots of bright and dark areas in the image or an improper environment like night time, the image captured by a camera may contain a lot of noise. Also, in cases of unwanted objects or colors in an image, these are also considered noise.
An example of salt and pepper noise looks like the following:

The preceding image can be easily generated using OpenCV as follows:
# initialize noise image with zeros
noise = np.zeros((400, 600))
# fill the image with random numbers in given range
cv2.randu(noise, 0, 256)
Let's add weighted noise to a grayscale image (on the left) so the resulting image will look like the one on the right:

The code for this is as follows:
# add noise to existing image
noisy_gray = gray + np.array(0.2*noise, dtype=np.int)
Here, 0.2 is used as parameter, increase or decrease the value to create different intensity noise.
In several applications, noise plays an important role in improving a system's capabilities, especially when we will use Deep Learning based models in the upcoming chapters. It is quite crucial for several applications, to know how robust the application is against noise becomes very important. As an example, we would want the model designed for applications like image classification to work with noisy images as well, hence noise is deliberately added in the images to test the application precision.