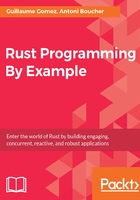
Playing with Options
To explain briefly how it works, when the Option type is Some, it simply means it contains a value whereas None doesn't. It has already been explained in Chapter 1, Basics of Rust, but here's a little recap just in case you need one. We can compare this mechanism with pointers in C-like languages; when the pointer is null, there is no data to access. The same goes for None.
Here's a short example:
fn pide(nb: u32, pider: u32) -> Option<u32> { if pider == 0 { None } else { Some(nb / pider) } }
So here, if the pider is 0, we can't pide or we'll get an error. Instead of setting an error or returning a complicated type, we just return an Option:
let x = pide(10, 3); let y = pide(10, 0);
Here, x is equal to Some(3) and y is equal to None.
The biggest advantage of this type compared to null is that if we have Some, you're sure that the data is valid. And in addition, when it's None, you can't accidentally read its content, it's simply impossible in Rust (and if you try to unwrap it, your program will panic immediately, but at least, you'll know what failed and why—no magical segmentation fault).
You can take a look at its documentation at https://doc.rust-lang.org/std/option/enum.Option.html.
Let's explain what happens here:
- We create a texture or return None if the creation fails.
- We set the color and then fulfill the texture with it.
- We return the texture.
If we return None, it simply means an error occurred. Also, for now, this function only handles two colors, but it's pretty easy to add more if you want.
It might look a bit complicated at the moment, but it'll make our life easier afterward. Now, let's call this function by creating a blue square of size 32x32:
let mut blue_square = create_texture_rect(&mut canvas, &texture_creator, TextureColor::Blue, TEXTURE_SIZE).expect("Failed to create a texture");
Easy, right?
Now we can just put pieces together. I'll let you try to handle the color switch. A small tip: take a look at the SystemTime struct. You can refer to its documentation at https://doc.rust-lang.org/stable/std/time/struct.SystemTime.html.