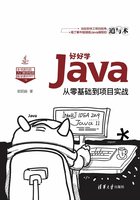
上QQ阅读APP看书,第一时间看更新
5.2.3 其他常见的字符串方法
无论是给字符串赋值,还是对字符串格式化,都属于往字符串填充内容,一旦内容填充完毕,就需进一步处理。譬如一段Word文本,常见的加工操作就有查找、替换、追加、截取等,按照字符串的处理结果异同,可将这些操作方法归为3大类,分别说明如下。
1.判断字符串是否具备某种特征
该类方法主要用来判断字符串是否满足某种条件,返回true代表条件满足,返回false代表条件不满足。判断方法的调用代码示例如下(完整代码见本章源码的src\com\string\string\StrMethod.java):
String hello="Hello World. 你好世界。"; boolean isEmpty=hello.isEmpty(); // isEmpty方法判断该字符串是否为空串 System.out.println("是否为空=" + isEmpty); boolean equals=hello.equals("你好"); //equals方法判断该字符串是否与目标串相等 System.out.println("是否等于你好=" + equals); boolean startsWith=hello.startsWith("Hello"); // startsWith方法判断该字符串是否以目标串开头 System.out.println("是否以Hello开头=" + startsWith); boolean endsWith=hello.endsWith("World"); // endsWith方法判断该字符串是否以目标串结尾 System.out.println("是否以World结尾=" + endsWith); boolean contains=hello.contains("or"); // contains方法判断该字符串是否包含目标串 System.out.println("是否包含or=" + contains);
运行以上的判断方法代码,得到以下的日志信息:
是否为空=false
是否等于你好=false
是否以Hello开头=true
是否以World结尾=false
是否包含or=true
2.在字符串内部根据条件定位
该类方法与字符串的长度有关,要么返回指定位置的字符,要么返回目标串的所在位置。定位方法的调用代码如下:
String hello="Hello World. 你好世界。"; int char_length=hello.length(); // length方法返回该字符串的字符数 System.out.println("字符数=" + char_length); int byte_length=hello.getBytes().length; // getBytes方法返回该字符串对应的字节数组 System.out.println("字节数=" + byte_length); char first=hello.charAt(0); // charAt方法返回该字符串在指定位置的字符 System.out.println("首字符=" + first); int index=hello.indexOf("l"); // indexOf方法返回目标串在源串第一次找到的位置 System.out.println("首次找到l的位置=" + index); int lastIndex=hello.lastIndexOf("l"); // lastIndexOf方法返回目标串在源串最后一次找到的位置 System.out.println("最后找到l的位置=" + lastIndex);
运行以上的定位方法代码,得到以下的日志信息:
字符数=18
字节数=28
首字符=H
首次找到l的位置=2
最后找到l的位置=9
3.根据某种规则修改字符串的内容
该类方法可对字符串进行局部或者全部的修改,并返回修改之后的新字符串。内容变更方法的调用代码示例如下:
String lowerCase=hello.toLowerCase(); // toLowerCase方法返回转换为小写字母的字符串 System.out.println("转换为小写字母=" + lowerCase); String upperCase=hello.toUpperCase(); // toUpperCase方法返回转换为大写字母的字符串 System.out.println("转换为大写字母=" + upperCase); String trim=hello.trim(); // trim方法返回去掉首尾空格后的字符串 System.out.println("去掉首尾空格=" + trim); String concat=hello.concat("Fine, thank you."); // concat方法返回在末尾添加目标串后的字符串 System.out.println("追加了目标串=" + concat); // 只有一个输入参数的substring方法,从指定位置一直截取到源串的末尾 String subToEnd=hello.substring(6); System.out.println("从第六位截取到末尾=" + subToEnd); // 有两个输入参数的substring方法,返回从开始位置到结束位置中间截取的子串 String subToCustom=hello.substring(6, 9); System.out.println("从第六位截取到第九位=" + subToCustom); String replace=hello.replace("l", "L"); // replace方法返回目标串替换后的字符串 System.out.println("把l替换为L=" + replace);
运行以上的内容变更方法代码,得到以下的日志信息:
转换为小写字母=hello world.你好世界。
转换为大写字母=HELLO WORLD.你好世界。
去掉首尾空格=Hello World.你好世界。
追加了目标串=Hello World.你好世界。Fine,thank you.
从第六位截取到末尾=World.你好世界。
从第六位截取到第九位=Wor
把l替换为L=HeLLo WorLd.你好世界。